Flux Redux Schnell
flux-redux-schnell
Flux Redux Schnell Model is a high-speed transformation model for efficient and precise image editing.
Model Information
Input
Configure model parameters
Output
View generated results
Result
Preview, share or download your results with a single click.
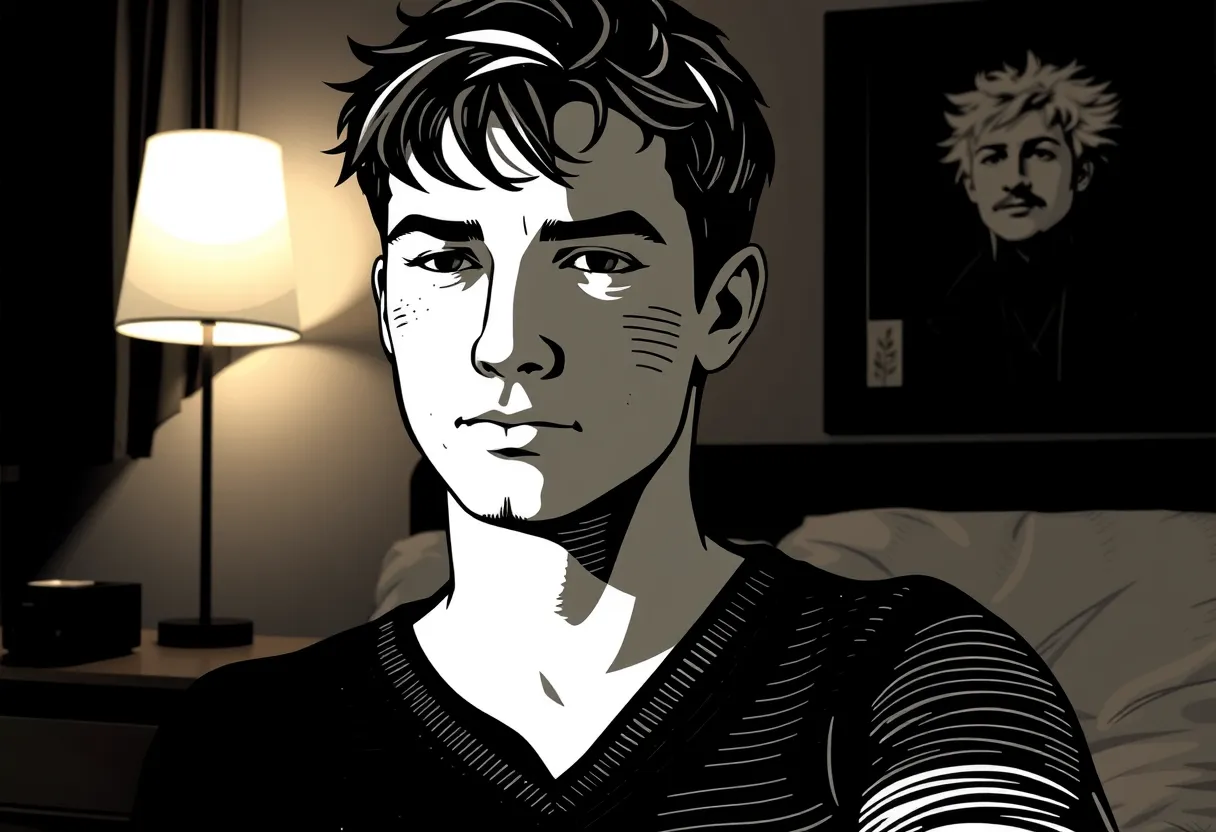
Prerequisites
- Create an API Key from the Eachlabs Console
- Install the required dependencies for your chosen language (e.g., requests for Python)
API Integration Steps
1. Create a Prediction
Send a POST request to create a new prediction. This will return a prediction ID that you'll use to check the result. The request should include your model inputs and API key.
import requestsimport timeAPI_KEY = "YOUR_API_KEY" # Replace with your API keyHEADERS = {"X-API-Key": API_KEY,"Content-Type": "application/json"}def create_prediction():response = requests.post("https://api.eachlabs.ai/v1/prediction/",headers=HEADERS,json={"model": "flux-redux-schnell","version": "0.0.1","input": {"seed": null,"megapixels": "1","num_outputs": "1","redux_image": "your_file.image/jpeg","aspect_ratio": "1:1","output_format": "webp","output_quality": "80","num_inference_steps": "4","disable_safety_checker": false}})prediction = response.json()if prediction["status"] != "success":raise Exception(f"Prediction failed: {prediction}")return prediction["predictionID"]
2. Get Prediction Result
Poll the prediction endpoint with the prediction ID until the result is ready. The API uses long-polling, so you'll need to repeatedly check until you receive a success status.
def get_prediction(prediction_id):while True:result = requests.get(f"https://api.eachlabs.ai/v1/prediction/{prediction_id}",headers=HEADERS).json()if result["status"] == "success":return resultelif result["status"] == "error":raise Exception(f"Prediction failed: {result}")time.sleep(1) # Wait before polling again
3. Complete Example
Here's a complete example that puts it all together, including error handling and result processing. This shows how to create a prediction and wait for the result in a production environment.
try:# Create predictionprediction_id = create_prediction()print(f"Prediction created: {prediction_id}")# Get resultresult = get_prediction(prediction_id)print(f"Output URL: {result['output']}")print(f"Processing time: {result['metrics']['predict_time']}s")except Exception as e:print(f"Error: {e}")
Additional Information
- The API uses a two-step process: create prediction and poll for results
- Response time: ~2 seconds
- Rate limit: 60 requests/minute
- Concurrent requests: 10 maximum
- Use long-polling to check prediction status until completion
Overview
Flux Redux Schnell is a versatile tool designed for generating detailed, high-quality outputs tailored to user-defined prompts and configurations. Its robust customization options allow users to produce visuals that align with their creative, professional, or exploratory needs. By adjusting various parameters, users can achieve a wide range of outputs, from abstract art to photorealistic renders.
Technical Specifications
- Advanced Customization: Offers fine control over outputs through adjustable parameters such as format, and quality.
- Image Input Support: Seamlessly process image-based inputs for enhanced usability.
- High-Resolution Outputs: Supports outputs at varying megapixel levels for diverse requirements.
Key Considerations
- Processing Time: Increasing num_inference_steps or output_quality will extend the generation time.
- Input Compatibility: Ensure the redux_image matches the intended style of the output.
- Safety Settings: Keep the safety checker enabled unless you are familiar with the input data and output requirements.
- Parameter Interactions: Test different combinations of aspect_ratio, megapixels, and output_quality to find the ideal configuration.
Legal Information
By using this model, you agree to:
- Black Forest Labs API agreement
- Black Forest Labs Terms of Service
Tips & Tricks
- Optimize Resolution: Start with medium megapixel for drafts, and switch to high resolution for final outputs.
- Blend Inputs: Combine a redux_image with a descriptive prompt for unique results.
- Aspect Ratio Framing: Choose an aspect ratio that complements the subject. For example, use 4:5 for portraits and 16:9 for cinematic scenes.
- Seed Experimentation: Adjust the seed value to explore creative possibilities, and lock it once satisfied.
- Prompt Crafting: Use descriptive and precise prompts to guide the model effectively. Combining visual and contextual details enhances output accuracy.
- Inference Steps: Increase num_inference_steps for more detailed and refined outputs, but note the trade-off with processing time.
- Output Quality: Adjust output_quality based on the use case. Higher quality is suited for final renders, while medium quality is optimal for drafts.
- Safety Checker: Disable the safety_checker only if you are confident about the safety of the input data.
Capabilities
- Generates outputs from textual, visual, or combined inputs.
- Supports a wide range of resolutions and aspect ratios.
- Flexible configurations for diverse artistic and professional use cases.
What can I use for?
- Creative Projects: Concept art, digital illustrations, and visual storytelling.
- Professional Applications: Branding, marketing visuals, and mockups.
- Exploration and Prototyping: Experiment with ideas and refine designs.
Things to be aware of
- Style Fusion: Input a redux_image and a contrasting prompt to blend styles.
- Resolution Scaling: Generate low-resolution drafts and upscale the best ones.
- Seed Consistency: Lock the seed to refine a specific output across iterations.
- Custom Aspect Ratios: Experiment with unique ratios to fit specific projects, like banners or profile images.
Limitations
- Abstract Prompts: The model may struggle with overly complex or vague prompts.
- High Parameters: Extreme values for num_inference_steps can result in slower processing times.
- Safety Checker: Disabling the checker may increase the risk of unintended outputs.
Output Format:WEBP,JPG,PNG