Flux Redux Dev
flux-redux-dev
Flux Redux Dev Model enables developers to perform advanced image transformations with custom results
Model Information
Input
Configure model parameters
Output
View generated results
Result
Preview, share or download your results with a single click.
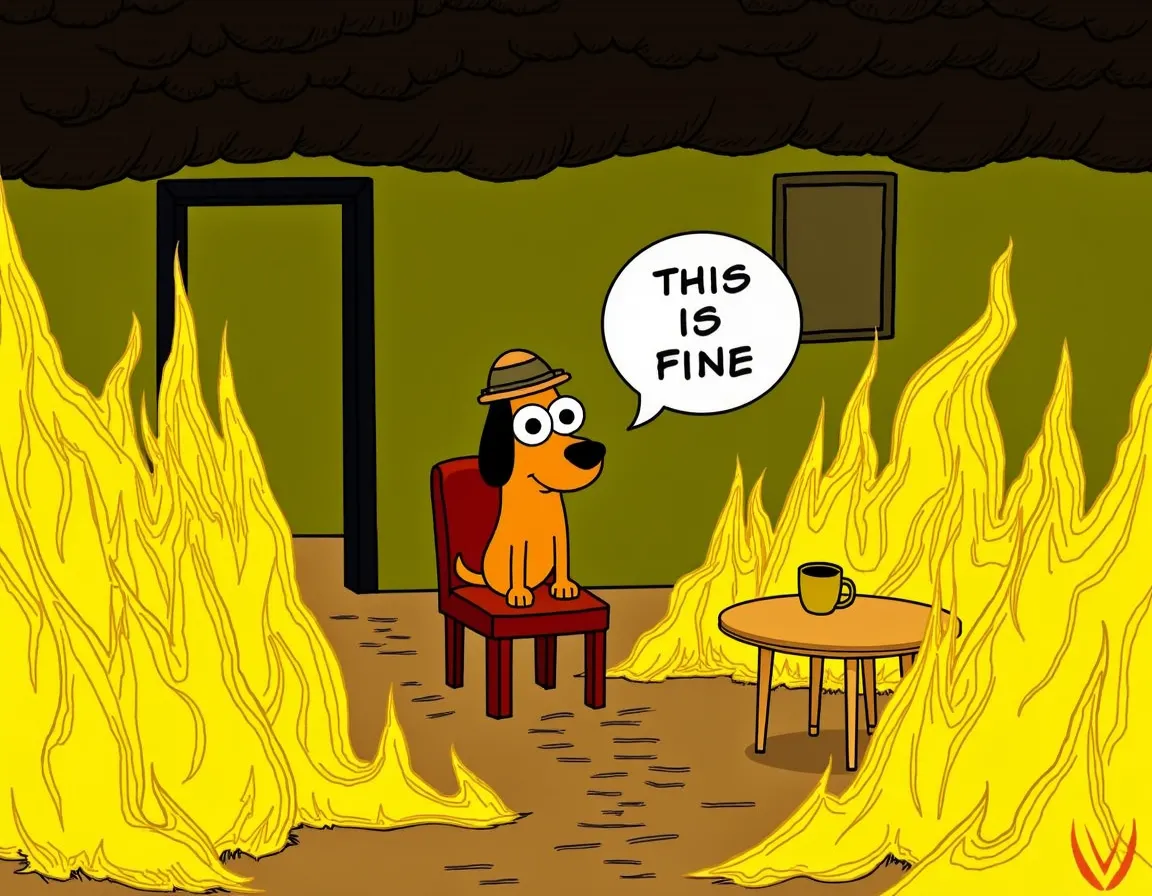
Prerequisites
- Create an API Key from the Eachlabs Console
- Install the required dependencies for your chosen language (e.g., requests for Python)
API Integration Steps
1. Create a Prediction
Send a POST request to create a new prediction. This will return a prediction ID that you'll use to check the result. The request should include your model inputs and API key.
import requestsimport timeAPI_KEY = "YOUR_API_KEY" # Replace with your API keyHEADERS = {"X-API-Key": API_KEY,"Content-Type": "application/json"}def create_prediction():response = requests.post("https://api.eachlabs.ai/v1/prediction/",headers=HEADERS,json={"model": "flux-redux-dev","version": "0.0.1","input": {"seed": 0,"guidance": 3,"megapixels": "1","num_outputs": 1,"redux_image": "your_file.image/jpeg","aspect_ratio": "1:1","output_format": "webp","output_quality": 80,"num_inference_steps": 28,"disable_safety_checker": false}})prediction = response.json()if prediction["status"] != "success":raise Exception(f"Prediction failed: {prediction}")return prediction["predictionID"]
2. Get Prediction Result
Poll the prediction endpoint with the prediction ID until the result is ready. The API uses long-polling, so you'll need to repeatedly check until you receive a success status.
def get_prediction(prediction_id):while True:result = requests.get(f"https://api.eachlabs.ai/v1/prediction/{prediction_id}",headers=HEADERS).json()if result["status"] == "success":return resultelif result["status"] == "error":raise Exception(f"Prediction failed: {result}")time.sleep(1) # Wait before polling again
3. Complete Example
Here's a complete example that puts it all together, including error handling and result processing. This shows how to create a prediction and wait for the result in a production environment.
try:# Create predictionprediction_id = create_prediction()print(f"Prediction created: {prediction_id}")# Get resultresult = get_prediction(prediction_id)print(f"Output URL: {result['output']}")print(f"Processing time: {result['metrics']['predict_time']}s")except Exception as e:print(f"Error: {e}")
Additional Information
- The API uses a two-step process: create prediction and poll for results
- Response time: ~7 seconds
- Rate limit: 60 requests/minute
- Concurrent requests: 10 maximum
- Use long-polling to check prediction status until completion
Overview
Flux Redux Dev is an open-weight image model developed by Black Forest Labs. It enables the creation of new versions of images while preserving key elements of the original. This model is particularly useful for tasks such as product photography, image editing, and content creation.
Technical Specifications
Model Architecture: Flux Redux Dev is built upon the FLUX.1 framework, designed for image variation tasks.
Input Requirements for Flux Redux Dev: Accepts images in standard formats with adjustable parameters for customization.
Output Capabilities: Generates high-quality image variations based on the provided input and parameters.
Key Considerations
- Content Sensitivity: Ensure that the input images comply with ethical guidelines and do not contain sensitive or inappropriate content.
- Resource Management: Be mindful of computational resources, especially when generating multiple high-resolution outputs.
- Parameter Tuning: Experiment with different parameter settings to achieve the desired balance between image quality and processing efficiency.
Legal Information
By using this Flux Redux Dev, you agree to:
- Black Forest Labs Privacy Policy
- Black Forest Labs Terms of Service
Tips & Tricks
- Aspect Ratio: Selecting an appropriate aspect ratio enhances the composition of the generated images. For example, 1:1 is ideal for portraits, while 16:9 suits landscapes.
- Number of Inference Steps: A higher number of inference steps (e.g., 40-50) can improve image quality but will increase processing time.
- Guidance Scale: A guidance scale between 7 and 8 often yields a good balance between adherence to the original image and introducing creative variations.
- Output Quality: For JPEG format, an output quality setting between 90 and 100 ensures high-quality images with minimal compression artifacts.
- Megapixels: Choosing a higher megapixel setting (e.g., 1) results in higher resolution images but requires more processing time and resources.
Capabilities
Image Variation: Generates diverse variations of the input image while maintaining core elements.
Customization: Offers adjustable parameters to fine-tune the output according to user preferences.
What can I use for?
- Image Variations – Generate multiple versions of an image while maintaining its core structure.
- Product Photography Enhancements – Modify lighting, colors, and textures while keeping the original product recognizable.
- Concept Art & Design Iterations – Create multiple artistic variations to explore different styles and compositions.
- Social Media Content – Adjust images for various platform aspect ratios and aesthetics.
- Photo Restoration & Improvement – Enhance image details and correct minor flaws while preserving realism.
Things to be aware of
Experiment with Aspect Ratios – Test different aspect ratios (e.g., 1:1 for social media, 16:9 for cinematic looks) to see how compositions change.
Optimize Inference Steps – Use a higher number of steps (e.g., 30–50) for detailed and refined outputs, or lower steps (e.g., 10–20) for faster results.
Adjust Guidance for Control – Set guidance between 3–7 for balanced creativity; higher values make the output more faithful to the original image.
Improve Image Sharpness with Output Quality – Increase output quality closer to 100 for high-fidelity results; reduce it to save processing time.
Test Different Megapixel Settings – Use 0.25 MP for quick previews and 1 MP for high-resolution outputs.
Limitations
Input Dependency: The quality of the output is heavily dependent on the quality of the input image.
Computational Load: Generating multiple high-resolution images can be resource-intensive and time-consuming.
Content Restrictions: The Flux Redux Dev may not perform well with images containing complex patterns or excessive noise.
Output Format: PNG,JPG,WEBP