SDXL Controlnet Lora
sdxl-controlnet-lora
SDXL Controlnet Lora is a model designed for controlled and high-quality image generation, combining SDXL features with precision editing.
Model Information
Input
Configure model parameters
Output
View generated results
Result
Preview, share or download your results with a single click.
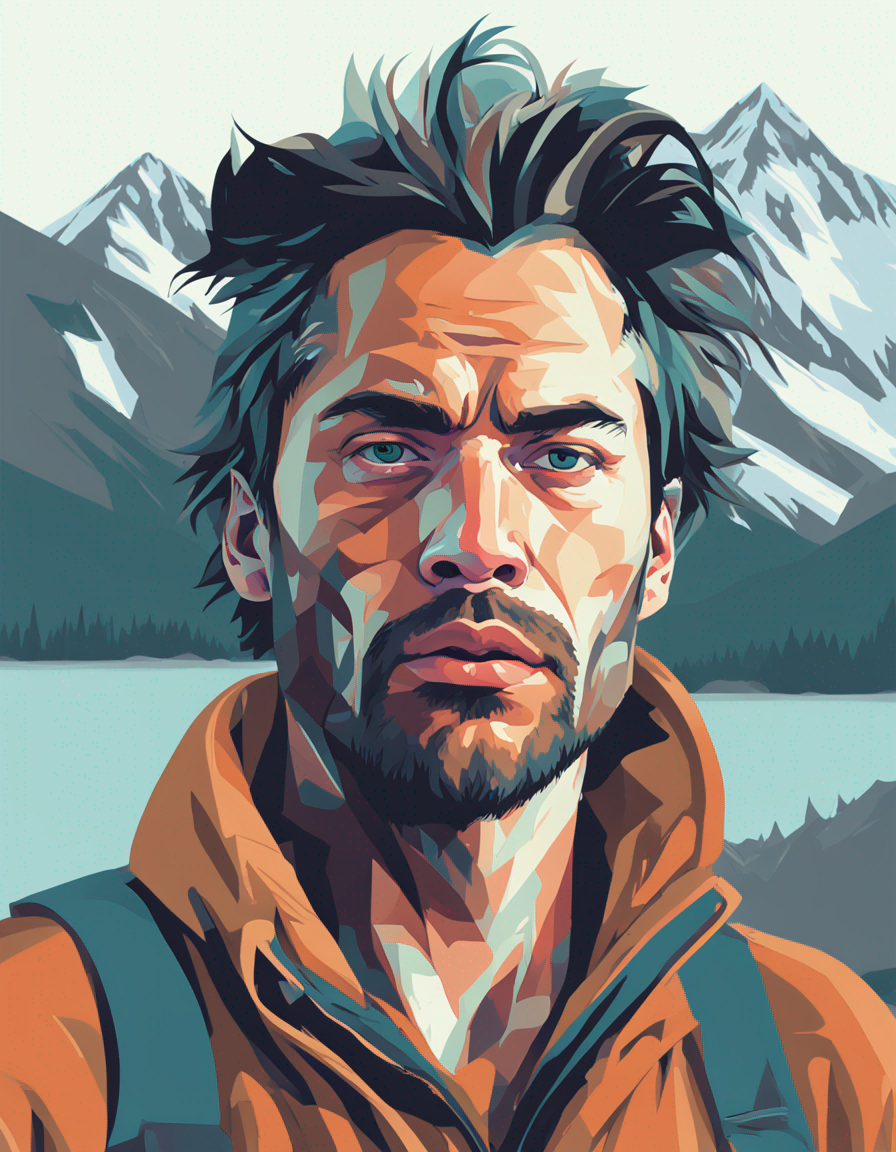
Prerequisites
- Create an API Key from the Eachlabs Console
- Install the required dependencies for your chosen language (e.g., requests for Python)
API Integration Steps
1. Create a Prediction
Send a POST request to create a new prediction. This will return a prediction ID that you'll use to check the result. The request should include your model inputs and API key.
import requestsimport timeAPI_KEY = "YOUR_API_KEY" # Replace with your API keyHEADERS = {"X-API-Key": API_KEY,"Content-Type": "application/json"}def create_prediction():response = requests.post("https://api.eachlabs.ai/v1/prediction/",headers=HEADERS,json={"model": "sdxl-controlnet-lora","version": "0.0.1","input": {"seed": 0,"image": "your_file.image/jpeg","prompt": "An astronaut riding a rainbow unicorn","refine": "base_image_refiner","img2img": false,"strength": 0.8,"scheduler": "K_EULER","lora_scale": 0.95,"num_outputs": 1,"lora_weights": "your lora weights here","refine_steps": 10,"guidance_scale": 7.5,"apply_watermark": false,"condition_scale": 1.1,"negative_prompt": "your negative prompt here","num_inference_steps": 30}})prediction = response.json()if prediction["status"] != "success":raise Exception(f"Prediction failed: {prediction}")return prediction["predictionID"]
2. Get Prediction Result
Poll the prediction endpoint with the prediction ID until the result is ready. The API uses long-polling, so you'll need to repeatedly check until you receive a success status.
def get_prediction(prediction_id):while True:result = requests.get(f"https://api.eachlabs.ai/v1/prediction/{prediction_id}",headers=HEADERS).json()if result["status"] == "success":return resultelif result["status"] == "error":raise Exception(f"Prediction failed: {result}")time.sleep(1) # Wait before polling again
3. Complete Example
Here's a complete example that puts it all together, including error handling and result processing. This shows how to create a prediction and wait for the result in a production environment.
try:# Create predictionprediction_id = create_prediction()print(f"Prediction created: {prediction_id}")# Get resultresult = get_prediction(prediction_id)print(f"Output URL: {result['output']}")print(f"Processing time: {result['metrics']['predict_time']}s")except Exception as e:print(f"Error: {e}")
Additional Information
- The API uses a two-step process: create prediction and poll for results
- Response time: ~17 seconds
- Rate limit: 60 requests/minute
- Concurrent requests: 10 maximum
- Use long-polling to check prediction status until completion
Overview
SDXL Controlnet Lora is designed to improve image generation by offering advanced control options through specific input settings. It lets users guide the image creation process using prompts, images, and fine-tuning parameters, allowing for more precise and customizable results. This makes it ideal for generating high-quality, visually appealing images that match detailed instructions and conditions.
Technical Specifications
Core Functionality for SDXL Controlnet Lora:
SDXL Controlnet Lora uses a combination of textual and visual inputs to guide image generation, incorporating advanced diffusion techniques and control mechanisms to produce tailored outputs.
Key Considerations
Input Quality:
- High-quality prompts and reference images yield better results. Avoid overly generic or unclear prompts.
- Use clear and relevant images for the image parameter to align the outputs effectively.
Parameter Settings:
- Overusing extreme values in parameters such as guidance_scale or lora_scale can lead to unnatural outputs.
- The balance between strength and condition_scale is crucial for effective conditioning.
Performance Impact:
Higher values for parameters like num_inference_steps or complex scheduler settings may increase processing time. Optimize these values based on desired output quality and available resources.
Tips & Tricks
- Prompt for SDXL Controlnet Lora:
Write descriptive and context-specific prompts. For example:- Instead of "a cat," use "a fluffy orange cat sitting on a wooden table in the sunlight."
- Image Reference:
Use high-resolution images with clear details. Avoid blurry or cluttered images as input. - Condition Scale:
- Recommended Value: 1.0 for balanced control; higher values make the control inputs more dominant, while lower values relax their influence.
- Strength:
- Recommended Value: 0.7–0.9 for img2img tasks. Lower values retain more of the original image details.
- Number of Inference Steps:
- Recommended Value: 50–150 for most tasks. Higher values improve detail but increase generation time.
- Scheduler:
- Options: DDIM, DPMSolverMultistep, HeunDiscrete, KarrasDPM, K_EULER_ANCESTRAL, K_EULER, PNDM.
- Suggested: Use KarrasDPM for smoother and more consistent outputs or DDIM for faster iterations.
- Guidance Scale:
- Recommended Value: 7–15 for balanced outputs. Higher values result in more prompt adherence but may reduce creativity.
- Refine:
- Options: no_refiner, base_image_refiner.
- Use base_image_refiner for additional post-processing to enhance details and sharpness.
- Lora Scale:
- Recommended Value: 0.5–0.8 for subtle fine-tuning. Higher values can oversaturate the effect of Lora weights.
- Negative Prompt:
- Use precise terms to exclude unwanted elements. For example, "blurry, low quality, oversaturated."
- Seed:
- Set a fixed value for reproducible results. Experiment with random values for diverse outputs.
- Watermark:
- Activate this for attribution or branding purposes if required.
Capabilities
SDXL Controlnet Lora transforms textual ideas into visually coherent images.
Modifying or enhancing existing images using the img2img functionality.
Fine-tuning outputs with Lora weights to align with specific artistic styles or requirements.
What can I use for?
Creative Projects with SDXL Controlnet Lora: Generate unique and visually stunning images based on descriptive prompts.
Concept Design: Develop early-stage concepts for visual arts, gaming, or animations.
Custom Styling: Apply fine-tuning to create outputs that align with specific artistic preferences.
Things to be aware of
Experiment with combining detailed prompts and high-quality images to guide generation.
Use scheduler options like KarrasDPM for smoother image generation.
Try different lora_scale values to balance fine-tuning effects.
Adjust negative_prompt to filter unwanted elements effectively.
Limitations
Overly complex prompts or excessive conditioning may lead to incoherent or overly stylized outputs.
img2img tasks are heavily influenced by the quality and relevance of the input image.
Higher guidance_scale or num_inference_steps values can increase processing time significantly.
Output Format: PNG